OpenID Connect (OIDC) is an authentication protocol layer that is built based on the Open Authorization (OAuth) 2.0 protocol. This topic describes how to obtain user information by using OIDC.
Prerequisites
A web application is created. The name, OAuth scopes, and callback URL are specified for the application. An application secret is created for the application. For more information, see Create an application, Add OAuth scopes, and Create an application secret.
Terms
Term | Description |
---|---|
ID token | OIDC can issue the ID token of a user to an application. The ID token can be used to obtain the user information, such as the username and logon name. The ID token cannot be used to access Alibaba Cloud resources. |
OIDC Discovery Endpoint | OIDC provides different endpoints for different purposes. Discovery Endpoint contains the necessary configuration information that is required by OIDC. Note Discovery Endpoint provides a set of keys in a JSON document. The keys contain information about the OIDC provider, such as the response type that is supported by OIDC, the value of the token issuer, the signing key endpoint of the ID token, and the signing algorithm. As an OIDC provider, Alibaba Cloud provides the following Discovery Endpoint to simplify the configuration: The following example shows the content of Discovery Endpoint:
|
Process
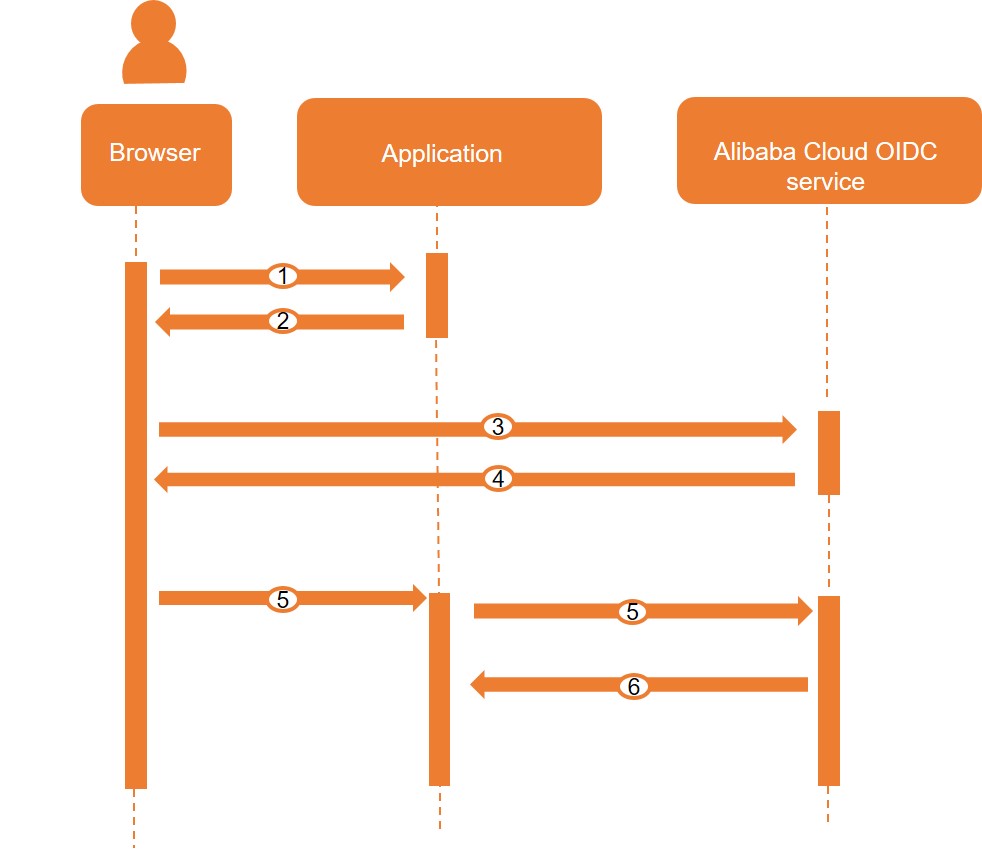
- A user logs on to an application by using a browser.
- The application redirects the user to the Alibaba Cloud OIDC service and sends the URL of the application to the browser. Note If the user is not logged on to Alibaba Cloud, the application redirects the user to the Alibaba Cloud logon page.
- The user logs on to the Alibaba Cloud OIDC service by using the browser and requests an authorization code.
- The Alibaba Cloud OIDC service returns an authorization code to the browser.
- The application requests an access token that corresponds to the user from the Alibaba Cloud OIDC service. The authorization code is required in the request.
- The Alibaba Cloud OIDC service returns the ID token and access token to the application. The application can use the ID token or access token to obtain user information.
- Parse the ID token to obtain user information.
The application must validate the signature of the ID token. The application obtains the signing key of the ID token, verifies the JSON Web Token (JWT) signature of the ID token, and then obtains the parsed user information. For more information, see Example 1: Obtain the signing key of an ID token, Example 2: Verify the JWT signature of an ID token, and Example 3: Parse an ID token to obtain user information.
- Use the ID token to communicate with different modules of the application.
The application must validate the signature of the ID token. The application obtains the signing key of the ID token and verifies the JWT signature of the ID token. For more information, see Example 1: Obtain the signing key of an ID token and Example 2: Verify the JWT signature of an ID token.
- Use the access token to query user information multiple times.
After the application obtains the access token, the application calls the UserInfo operation to obtain user information. For more information, see Example 4: Obtain user information by using an access token and the UserInfo operation.
- Parse the ID token to obtain user information.
Example 1: Obtain the signing key of an ID token
Sample request
private List getSignPublicKey() {
HttpResponse response = HttpClientUtils.doGet("https://oauth.alibabacloud.com/v1/keys");
List rsaKeyList = new ArrayList();
if (response.getCode() == 200 && response.isSuccess()) {
String keys = JSON.parseObject(response.getData()).getString("keys");
try {
JSONArray publicKeyList = JSON.parseArray(keys);
for (Object object : publicKeyList) {
RSAKey rsaKey = RSAKey.parse(JSONObject.toJSONString(object));
rsaKeyList.add(rsaKey);
}
return rsaKeyList;
} catch (Exception e) {
LOG.info(e.getMessage());
}
}
LOG.info("GetSignPublicKey failed:{}", response.getData());
throw new AuthenticationException(response.getData());
}
Example 2: Verify the JWT signature of an ID token
- Token signature: Alibaba Cloud checks whether the ID token is valid by using the public signing key that is issued by OAuth.
Sample request
public boolean verifySign(SignedJWT signedJWT) { List publicKeyList = getSignPublicKey(); RSAKey rsaKey = null; for (RSAKey key : publicKeyList) { if (signedJWT.getHeader().getKeyID().equals(key.getKeyID())) { rsaKey = key; } } if (rsaKey != null) { try { RSASSAVerifier verifier = new RSASSAVerifier(rsaKey.toRSAPublicKey()); if (signedJWT.verify(verifier)) { return true; } } catch (Exception e) { LOG.info("Verify exception:{}", e.getMessage()); } } throw new AuthenticationException("Can't verify signature for id token"); }
- Token validity: Alibaba Cloud checks the time when the ID token was issued and the validity period of the ID token.
- Token recipient: Alibaba Cloud prevents ID tokens that are issued to other applications from being issued to the application.
Example 3: Parse an ID token to obtain user information
- Response parameters
- Header parameters
Parameter Description Required OAuth scope alg The signing algorithm. openid kid The public key that is used to validate the ID token. You must use this key to validate your signature and prevent your ID token from being tampered with. openid - Body parameters
Parameter Description Required OAuth scope exp The timestamp when the token expires. openid sub A unique string that indicates the user. The string does not contain the UID and username of the user. openid aud The receiver of the token, which is the ID of the OAuth application. openid iss The issuer of the token. The value is https://oauth.alibabacloud.com. openid iat The timestamp when the token was issued. openid name The display name of the user. profile upn The logon name of the Resource Access Management (RAM) user. This parameter is returned only when a RAM user sends the request. profile login_name The logon name of the Alibaba Cloud account. This parameter is returned only when an Alibaba Cloud account sends the request. profile aid The ID of the Alibaba Cloud account to which the user belongs. aliuid uid The ID of the user. If you log on to Alibaba Cloud with an Alibaba Cloud account, the value is the ID of the Alibaba Cloud account. In this case, the value of this parameter is the same as the value of the aid parameter. If you log on to Alibaba Cloud as a RAM user, the value is the ID of the RAM user. aliuid
- Header parameters
- Sample responses
- Sample response header
{ "alg": "RS256", "kid": "JC9wxzrhqJ0gtaCEt2QLUfevEUIwltFhui4O1bh****" }
- Sample response body
For demonstration purposes, the following sample responses show an ID token before encoding. In actual scenarios, an encoded ID token is returned. For more information, see Example 2: Verify the JWT signature of an ID token.
- Sample response body of an Alibaba Cloud account-initiated request
{ "exp": 1517539523, "sub": "123456789012****", "aud": "4567890123456****", "iss": "https://oauth.alibabacloud.com", "iat": 1517535923, "name": "alice", //The display name of the user. "login_name":"alice@example.com", //The logon name of the Alibaba Cloud account. "aid": "123456789012****", //The ID of the Alibaba Cloud account. "uid": "123456789012****" //The ID of the Alibaba Cloud account. }
- Sample response body of a RAM user-initiated request
{ "exp": 1517539523, "sub": "123456789012****", "aud": "4567890123456****", "iss": "https://oauth.alibabacloud.com", "iat": 1517535923, "name": "alice", //The display name of the user. "upn": "alice@example.onaliyun.com", //The logon name of the RAM user. "aid": "123456789012****", //The ID of the Alibaba Cloud account to which the RAM user belongs. "uid": "234567890123****" //The ID of the RAM user. }
- Sample response body of an Alibaba Cloud account-initiated request
- Sample response header
Example 4: Obtain user information by using an access token and the UserInfo operation
After you obtain an access token, you can call the UserInfo operation to obtain user information. The UserInfo operation can be called only by using an access token, and the response information is not encoded.
The endpoint for the UserInfo operation is https://oauth.alibabacloud.com/v1/userinfo
.
Sample request
GET v1/userinfo HTTP/1.1
Host: oauth.alibabacloud.com
Authorization: Bearer SIAV32hkKG
Response parameters
Parameter | Description | Required OAuth scope |
---|---|---|
sub | A unique string that indicates the user. The string does not contain the UID and username of the user. | openid |
name | The display name of the user. | profile |
upn | The logon name of the RAM user. This parameter is returned only when a RAM user sends the request. | profile |
login_name | The logon name of the Alibaba Cloud account. This parameter is returned only when an Alibaba Cloud account sends the request. | profile |
aid | The ID of the Alibaba Cloud account to which the user belongs. | aliuid |
uid | The ID of the user. If you log on to Alibaba Cloud with an Alibaba Cloud account, the value is the ID of the Alibaba Cloud account. In this case, the value of this parameter is the same as the value of the aid parameter. If you log on to Alibaba Cloud as a RAM user, the value is the ID of the RAM user. | aliuid |
Sample response body
- Sample response body of an Alibaba Cloud account request
HTTP/1.1 200 OK Content-Type: application/json { "sub": "123456789012****", "name": "alice", //The display name of the user. "login_name":"alice@example.com", //The logon name of the Alibaba Cloud account. "aid": "123456789012****", //The ID of the Alibaba Cloud account. "uid": "123456789012****" //The ID of the Alibaba Cloud account. }
- Sample response body of a RAM user request
HTTP/1.1 200 OK Content-Type: application/json { "sub": "123456789012****", "name": "alice", //The display name of the user. "upn": "alice@example.onaliyun.com", //The logon name of the RAM user. "aid": "123456789012****", //The ID of the Alibaba Cloud account to which the RAM user belongs. "uid": "234567890123****" //The ID of the RAM user. }