This topic describes how frontend JavaScript web applications access Drive and Photo Service by using OAuth 2.0. Frontend JavaScript web applications can be Chrome plug-ins or widgets in JavaScript.
Because the applications are frontend applications, it is not secure to store confidential information such as AppSecrets in these applications. The procedure of Drive and Photo Service authorization for these applications is different from that for web server applications.
1. Overview
(1) OAuth 2.0 Implicit Grant Type
Implicit Grant Type is a way to directly obtain an access token from the authorization server of a browser, without the need to obtain an authorization code from a third-party application server. All the operations are performed in the browser. The token is visible to all visitors and does not require authorization on the client side.
(2) Flowchart of an SPA
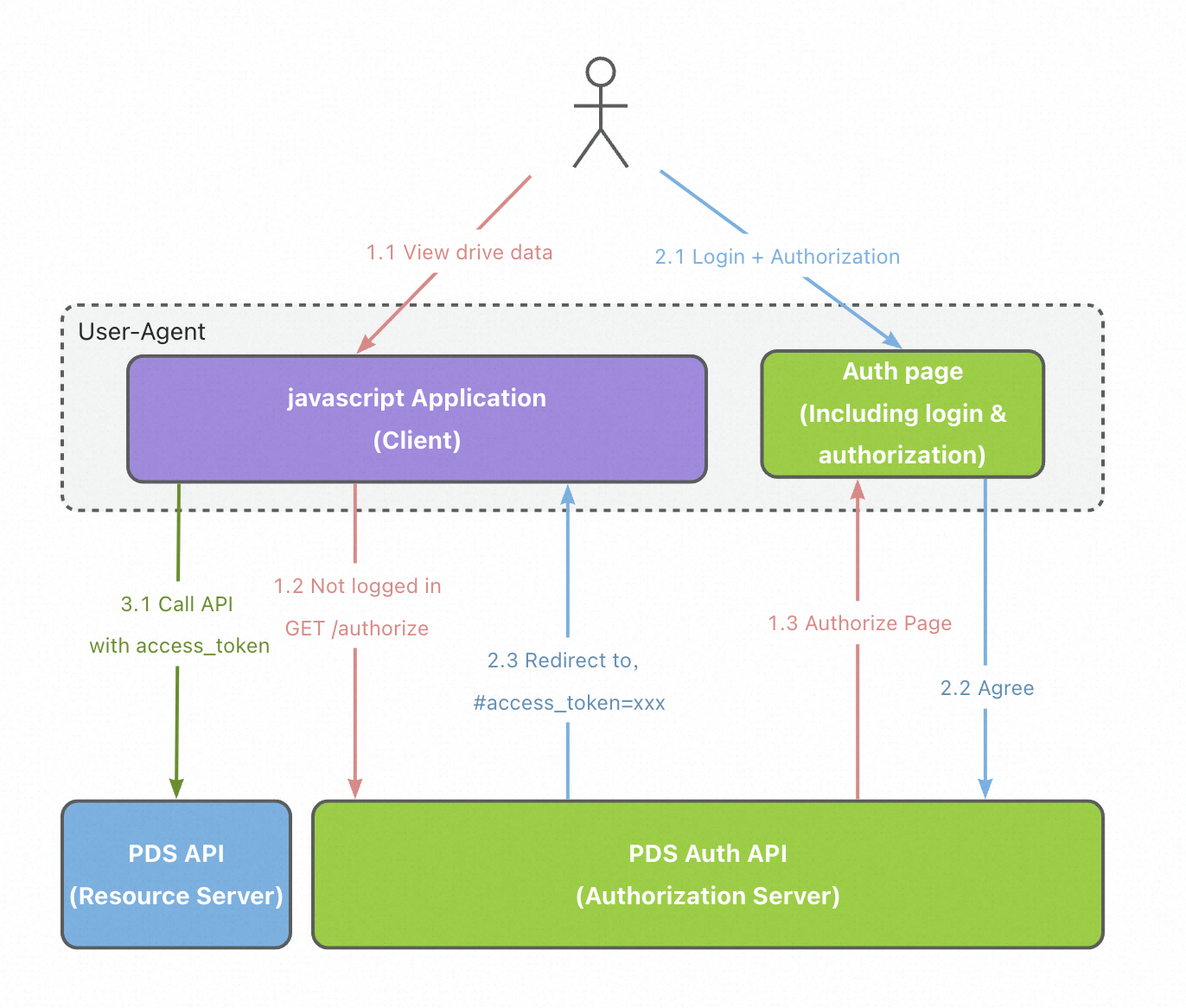
2. Preparations
(1) Create a domain
Create a domain in the PDS (Drive and Photo Service) console. After a domain is created, a fourth-level API domain name in the format of https://{domainId}.api.aliyunpds.com
is provided.
(2) Enable the logon page
Enable the logon page that is provided by Drive and Photo Service for authorization. Drive and Photo Service provides the following fourth-level domain name: https://{domainId}.api.aliyunpds.com
.
(3) Create an application to be used as an OAuth client in the Drive and Photo Service console
Create an application. Select WebBrowser (Web Client Application) as Type. Specify the Permission Scope parameter. For more information, see Scopes. The scopes will be displayed on the consent page. Configure a redirect URI. After the application is created, you can obtain the AppId of the application. The AppId is used as the ClientId for OAuth authorization.
3. Authorization principle and API operations
(1) Call the Authorization operation
Run the following commands on a new page:
// Open a web page for authorization.
var domainId ='Your domain ID'
var authWin = window.open('https://'+domainId+'.api.aliyunpds.com/v2/oauth/authorize?client_id='+APP_ID+'&response_type=token&state=abc&login_type=default&redirect_uri=http://example.com/callback', '_blank', 'location=0,status=0,titlebar=0,menubar=0,resizable=0,height=500,width=600', true)
authWin.onmessage=function(e){
var hash = e.data;
// Parse the value of hash to obtain the access token.
}
API request syntax:
GET /v2/oauth/authorize?client_id=<APPID>&response_type=token&state=[state]&login_type=<login_type>&redirect_uri=<redirect_uri> HTTP/1.1
Host: {domainId}.api.aliyunpds.com
Parameter | Required | Description |
client_id | Yes | The AppId of your application. If you do not have an AppId, create an application to obtain an AppId in the Drive and Photo Service console. |
redirect_uri | Yes | The URI to which the Drive and Photo Service authorization server redirects the user after the authorization process is complete. A URL that is provided by the web server application. Example: |
scope | No | The scopes that specify the permissions required by your application. The scopes will be displayed on the consent page. For more information, see Scopes. |
response_type | Yes | The type of the response. Set the value to |
state | No, but recommended | If this parameter is specified, the Drive and Photo Service authorization server returns this value intact in the redirect URI to prevent replay attacks. |
login_type | Yes | The logon method. Valid values: |
hide_consent | No | Specifies whether to display the consent page if the user logs on for the first time. Valid values: |
lang | No | The language in which the page is displayed. Valid values: |
(2) Redirect URIs
If the authorization is successful, the Drive and Photo Service authorization server redirects users to a URI in the following format:
http://example.com/callback#access_token=xxx&expires_in=xxx&token_type=Bearer
If the authorization fails, the Drive and Photo Service authorization server redirects users to a URI in the following format:
http://example.com/callback#error=xxxxxx
Sample code:
<!DOCTYPE html>
<html>
<body>
<script>
var hash = location.hash;
if (hash) {
var sch = new URLSearchParams(hash.replace(/^#?/g, ''))
var access_token = sch.get('access_token')
var expires_in = parseInt(sch.get('expires_in'))
var expire_time = sch.get('expire_time')
if(!isNaN(expires_in) && !expire_time){
expire_time=new Date(Date.now()+expires_in*1000).toISOString()
}
var token_type = sch.get('token_type')
var state = JSON.parse(sch.get('state') || '{}')
top.opener.postMessage({ access_token, expires_in,expire_time, token_type }, state.origin)
window.location.replace(window.location.origin + window.location.pathname)
} else {
window.close()
}
</script>
</body>
</html>
Parse the value of hash on the callback page to obtain the access token. Use postMessage() to enable communication between the web page for authentication and the page to which users are redirected.
(3) Obtain an access token
On the web page for authentication, you can obtain the access token that is returned by postMessage() from the redirected page.
4. Call Drive and Photo Service API operations
The application can use the access token to call Drive and Photo Service API operations. The access token must be included in the Authorization header of API requests.
For more information, see Invoking Methods.