The short video SDK provides the basic recording feature and allows you to apply recording effects such as background music, speed ramping, and face stickers to short videos.
Supported editions
Edition | Supported |
---|---|
Professional | Yes |
Standard | Yes |
Basic | Yes |
Related classes
Class | Description |
---|---|
AliyunIRecorder | A core class that defines recording features, including recording, preview settings, effect settings, and callback settings. |
AliyunClipManager | A class that manages video clips, for example, obtains the clip information or deletes video clips. |
AliyunIRecorderDelegate | A class that defines recording proxy callbacks. |
Configure recording
The following figure shows the process for basic recording:
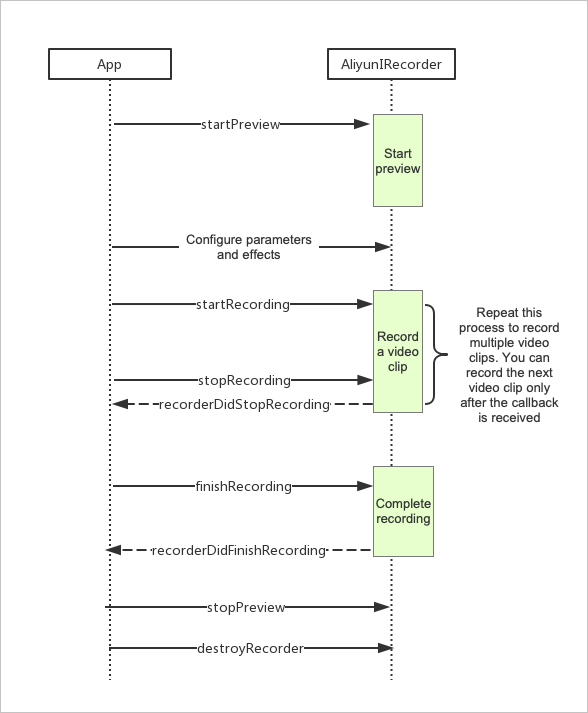
Configuration | Step | Description | Sample code |
---|---|---|---|
Basic configurations | 1 | Initialize the AliyunIRecorder class, create a recording instance, and configure recording parameters. | Initialize the class |
2 | Start and stop preview. | Configure preview | |
3 | Start, cancel, and stop recording a video clip. | Start recording | |
4 | Stop recording and generate configuration information. | Stop recording | |
Advanced configurations | 5 | Configure parameters such as the maximum or minimum recording duration, how to delete a video clip, and how to query the number of video clips. Configure these parameters based on your business requirements. | Manage video clips |
6 | Configure recording effects such as retouching, filters, and background music based on your business requirements. | Configure effects | |
7 | Configure common callbacks and how to handle special events such as screen lock, incoming call, and switching to the background. | Handle events |
Initialize the class
Initialize the AliyunIRecorder class, create a recording instance, and configure recording parameters. For more information about the parameters that are used in the code, see Related classes.
- taskPath specifies the path of the folder where recording-related configurations are stored.
- The aspect ratio of the preview must be the same as the value of videoSize.
CGSize resolution = CGSizeMake(720, 1280); // 720P
AliyunIRecorder *recorder = [[AliyunIRecorder alloc] initWithDelegate:self videoSize:resolution];
recorder.taskPath = taskPath;// Specify the path of the folder.
recorder.preview = self.videoView;// // Configure the preview view.
recorder.recordFps = 30;
recorder.GOP = recorder.recordFps * 3; // Set the keyframe interval to 3 seconds.
recorder.outputPath = [taskPath stringByAppendingPathComponent:@"output.mp4"];// Specify the output path of the recorded video.
recorder.frontCaptureSessionPreset = AVCaptureSessionPreset1280x720;
recorder.backCaptureSessionPreset = AVCaptureSessionPreset1280x720;
// Configure video clips.
recorder.clipManager.deleteVideoClipsOnExit = YES; // All video clips are automatically deleted when you exit the application. Alternatively, you can delete taskPath after the recording is complete.
recorder.clipManager.maxDuration = 15;
recorder.clipManager.minDuration = 3;
self.aliyunRecorder = recorder;
Configure preview
For more information about the parameters that are used in the code, see Related classes.
// Call AliyunIRecorderCameraPositionFront to enable preview for the front camera.
// Call AliyunIRecorderCameraPositionBack to enable preview for the rear camera.
[self.aliyunRecorder startPreviewWithPositon:AliyunIRecorderCameraPositionFront];
Configure preview parameters
// Turn on the flashlight. You can turn on the flashlight when the rear camera is used.
AliyunIRecorderTorchMode torchMode = self.aliyunRecorder.cameraPosition == AliyunIRecorderCameraPositionBack ? AliyunIRecorderTorchModeOn : AliyunIRecorderTorchModeOff;
[self.aliyunRecorder switchTorchWithMode:torchMode];
// Switch between the front and rear cameras.
[self.aliyunRecorder switchCameraPosition];
// Specify the zoom factor.
self.aliyunRecorder.videoZoomFactor = 30.0;
// Specify the camera angle.
self.aliyunRecorder.cameraRotate = 270;
// Specify the video resolution.
[self.aliyunRecorder reStartPreviewWithVideoSize:CGSizeMake(720, 720)];
Stop preview
[self.aliyunRecorder stopPreview];
Start recording
startRecording and stopRecording must be called in pairs. You can call them once or multiple times, and one or more temporary video clips are generated. For more information about the parameters that are used in the code, see Related classes.
// Start recording a video clip.
[self.aliyunRecorder startRecording];
// Stop recording a video clip.
[self.aliyunRecorder stopRecording];
Stop recording
- finishRecording: merges the recorded video clips into one video. Call this API operation if you do not need to continue editing the generated video.
- finishRecordingForEdit: does not merge the recorded video clips. Call this API operation if you want to continue editing the generated video later. You can initialize the editor by configuring the taskPath parameter. For more information, see Initialize an editor.
// Stop recording and merge the recorded video clips into one video.
[self.aliyunRecorder finishRecording];
// Stop recording without merging the recorded video clips into one video. taskPath is generated.
NSString *taskPath = [self.aliyunRecorder finishRecordingForEdit];
AliyunEditor *editor = [[AliyunEditor alloc] initWithPath:taskPath preview:preview];
...
Manage video clips
Configure parameters such as the maximum or minimum recording duration, how to delete a video clip, and how to query the number of video clips. Configure these parameters based on your business requirements. For more information about the parameters that are used in the code, see Related classes.
// Delete the last video clip.
[self.aliyunRecorder.clipManager deletePart];
// Delete all video clips.
[self.aliyunRecorder.clipManager deleteALLPart];
// Obtain the total number of video clips.
[self.aliyunRecorder.clipManager partCount];
Configure effects
Configure recording effects such as retouching, filters, and background music based on your business requirements. For more information about the parameters that are used in the code, see Related classes.
Filters
// Add a filter.
NSString *filterDir = [self.class resourcePath:@"Filter/Jiaopian"];
AliyunEffectFilter *filter = [[AliyunEffectFilter alloc] initWithFile:filterDir];
[self.aliyunRecorder applyFilter:filter];
// Remove a filter.
[self.aliyunRecorder deleteFilter];
// Add an animated filter.
NSString *filterDir = [self.class resourcePath:@"AnimationEffect/split_screen_3"];
AliyunEffectFilter *animationFilter =[[AliyunEffectFilter alloc] initWithFile:filterDir];
[self.aliyunRecorder applyAnimationFilter:animationFilter];
// Remove an animated filter.
[self.aliyunRecorder deleteAnimationFilter];
Face stickers
// Use the built-in facial recognition feature to add face stickers.
self.aliyunRecorder.useFaceDetect = YES;// Enable facial recognition. Faces can be automatically tracked.
self.aliyunRecorder.faceDetectCount = 3;// Specify the number of faces that can be recognized. Maximum value: 3. Minimum value: 1. If you do not need facial recognition, set the useFaceDetect parameter to NO.
self.aliyunRecorder.faceDectectSync = YES;// Allow face stickers to fit into faces in real time. Face stickers can fit well into moving faces. However, stuttering may occur on devices with poor performance. If you do not enable this feature, though the smoothness of videos can be ensured, face stickers badly fit into moving faces.
NSString *parsterDir = [self.class resourcePath:@"Gif/hanfumei-800"];
AliyunEffectPaster *paster = [[AliyunEffectPaster alloc] initWithFile:parsterDir];
[self.aliyunRecorder applyPaster:paster];// Add a face sticker.
// Remove a face sticker.
[self.aliyunRecorder deletePaster:paster];
// Disable the built-in facial recognition feature.
self.aliyunRecorder.useFaceDetect = NO;
// Call the facial recognition library of a third party in the callback to recognize faces. Results are returned.
- (void)recorderOutputVideoRawSampleBuffer:(CMSampleBufferRef)sampleBuffer {
NSArray<AliyunFacePoint *> *facePoints = ...; // Use the facial recognition library of a third party to recognize faces.
if (self.aliyunRecorder.faceNumbersCallback) {
int num = (int)facePoints.count;
self.aliyunRecorder.faceNumbersCallback(num);
}
[self.aliyunRecorder faceTrack:facePoints];
}
Watermarks
// Add a watermark.
NSString *watermarkPath = [self.class resourcePath:@"Image/watermark.png"];
AliyunEffectImage *watermark = [[AliyunEffectImage alloc] initWithFile:watermarkPath];
CGSize size = CGSizeMake(280, 200);
CGFloat centerx = 44 + size.width / 2.0;
CGFloat centery = 44 + size.height / 2.0;
watermark.frame = CGRectMake(centerx, centery, size.width, size.height); // Take note that the origin of a frame indicates the center point.
[self.aliyunRecorder applyImage:watermark];
// Remove a watermark.
[self.aliyunRecorder deleteImage:watermark];
// Add music.
AliyunEffectMusic *effectMusic =[[AliyunEffectMusic alloc] initWithFile:[self.class resourcePath:@"bgm.aac"]];
effectMusic.startTime = 3.0; // The music plays from the 3-second position.
effectMusic.duration = 5.0; // The music plays for 5 seconds. If you do not configure this parameter, the music plays for its remaining duration of up to 360 seconds.
[self.aliyunRecorder applyMusic:effectMusic];
// Remove music.
[self.aliyunRecorder applyMusic:nil];
Advanced retouching
- Built-in retouching
// Enable built-in retouching. self.aliyunRecorder.beautifyStatus = YES; self.aliyunRecorder.beautifyValue = 80; // Disable built-in retouching. self.aliyunRecorder.beautifyStatus = NO; self.aliyunRecorder.beautifyValue = 0;
- Retouching SDKs
To use effects provided by a retouching SDK in the short video SDK, you must first obtain the permissions on the retouching SDK and integrate the retouching SDK into the short video SDK.
- For more information about how to integrate Alibaba Cloud Queen SDK, see Queen SDK. For more information about the sample code for configuring effects, see Sample code.
- For more information about how to purchase, integrate, and use FaceUnity, see FaceUnity.
The short video SDK passes the CMSampleBufferRef data that is collected by the camera to the business layer by using AliyunIRecorderDelegate. The retouching SDK that is imported into the business layer performs custom rendering of the data and then returns the rendering result CVPixelBufferRef to the short video SDK for preview and production. Take note that the retouching SDK conflicts with the GL environment of the short video SDK.
// AliyunIRecorderDelegate - (CVPixelBufferRef)customRenderedPixelBufferWithRawSampleBuffer:(CMSampleBufferRef)sampleBuffer { if (!self.enableBeauty) { return CMSampleBufferGetImageBuffer(sampleBuffer); // This is returned if you do not perform custom rendering. } // If you integrate Queen SDK to perform custom rendering, sample CVPixelBufferRef is returned. ... }
// Take a photo and asynchronously obtain the following types of images:
// image: the rendered image that is collected.
// rawImage: the raw image that is collected.
[self.aliyunRecorder takePhoto:^(UIImage *image, UIImage *rawImage) {
}];
Handle events
Configure common callbacks and how to handle special events such as screen lock, incoming call, and switching to the background. For more information about the parameters that are used in the code, see Related classes.
Callbacks
// Configure common callbacks as follows:
- (void)recorderDeviceAuthorization:(AliyunIRecorderDeviceAuthor)status {
dispatch_async(dispatch_get_main_queue(), ^{
if (status == AliyunIRecorderDeviceAuthorAudioDenied) {
[DeviceAuthorization openSetting:@"You are not authorized to use the microphone."];
} else if (status == AliyunIRecorderDeviceAuthorVideoDenied) {
[DeviceAuthorization openSetting:@"You are not authorized to use the camera."];
}
});
}
- (void)recorderVideoDuration:(CGFloat)duration {
// Update the recording progress.
NSLog(@"Record Video Duration: %f", duration);
}
- (void)recorderDidStopRecording {
NSLog(@"Record Stop Recording");
if (self.recordState == RecorderStateFinish) {
if (self.aliyunRecorder.clipManager.duration >= self.aliyunRecorder.clipManager.minDuration) {
[self.aliyunRecorder finishRecording];
}
else {
self.recordState = RecorderStatePreviewing;
}
}
}
- (void)recorderWillStopWithMaxDuration {
NSLog(@"Record Will Stop Recording With Max Duration");
[self.aliyunRecorder stopPreview];
}
- (void)recorderDidStopWithMaxDuration {
NSLog(@"Record Did Recording With Max Duration");
[self.aliyunRecorder finishRecording];
}
- (void)recorderDidFinishRecording {
NSLog(@"Record Did Finish Recording");
[self.aliyunRecorder stopPreview];
self.recordState = RecorderStateInit;
}
- (void)recoderError:(NSError *)error {
NSLog(@"Record Occurs Error: %@", error);
}
Other events
Special events include screen lock, incoming call, and switching to the background. You must listen to the UIApplicationWillResignActiveNotification event. Before the application enters the Inactive state, call the stopRecording and stopPreview methods to stop the preview. You must also listen to the UIApplicationDidBecomeActiveNotification event. After the application enters the Active state, call the startPreview method to restart the preview.