AUNavigationBar is a navigation bar control of mPaaS. It extends the UINavigationBar control and provides default mPaaS navigation bar styles. To facilitate subsequent extension, use AUNavigationBar rather than UINavigationBar in all mPaaS apps.
Sample image
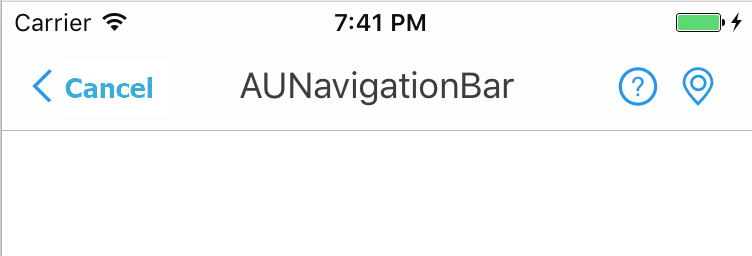
API description
/**
The mPaaS navigation bar control that contains mPaaS navigation bar styles.
Initialize:
UINavigationController *navBar = [[UINavigationController alloc] initWithNavigationBarClass:NSClassFromString(@"AUNavigationBar") toolbarClass:nil];
*/
@interface AUNavigationBar : UINavigationBar
@end
/**
The UINavigationBar extension that defines default UINavigationBar styles.
*/
@interface UINavigationBar (AUNavigationBarExtensions)
/**
* Return the default color of the title of framework navigation bar. The default value is #000000.
*
* @return
*/
+ (UIColor*)getNavigationBarTitleDefaultColor;
/**
* Return the color of the item of framework navigation bar. The default value is #108EE9.
*
* @return
*/
+ (UIColor*)getNavigationBarButtonItemDefaultColor;
/**
* Return the color of the item of framework navigation bar. The default value is #fffff.
*
* @return
*/
+ (UIColor*)getNavigationBarDefaultColor;
/**
* Get the color of the bottom line of the navigation bar. The default value is #e1e1e1.
*
* @return
*/
+ (UIColor*)getNavigationBarBotLineColor;
/**
* Note:
* 1. The base class DTViewController sets the default style of navigation bar in ViewWillAppear.
* 2. Business personnel can call the system APIs or the following APIs to change the style of navigation bar. The style is typically set in ViewWillAppear.
* 3. If VC is a subclass of DTViewController, it must be set in ViewWillAppear, or it will be overridden.
* 4. Ensure that setNavigationBarDefaultStyle is used to restore the default style when ViewWillDisappear is called after the modification.
* 5. If VC defines the homepage in the UITabBarController container, do not use setNavigationBarDefaultStyle to restore the default style, or the VC will be overridden upon tab switching.
*/
/**
*
* Set the background of default navigation bar. The default background color and bottom line color are #ffffff and #e1e1e1, respectively.
*
*/
- (void)setNavigationBarDefaultStyle;
/**
*
* Set the default title style of the navigation bar.
*
*/
- (void)setNavigationBarDefaultTitleTextAttributes;
/**
*
* Set the title color of the navigation bar in ViewWillAppear, or the title color will be overridden by the default title color in the framework.
*
*/
- (void)setNavigationBarTitleTextAttributesWithTextColor:(UIColor *)textColor;
/**
*
* Set the transparency of the navigation bar.
* Note: If this method sets the navigation bar to be completely transparent, returned animation will flash white. Currently, this issue has not been resolved. Do not call this method. If the method is required, evaluate whether the impact is acceptable.
*/
- (void)setNavigationBarTranslucentStyle;
/**
* Set the color of the navigation bar. To achieve the frosted glass effect, set translucent to Yes.
* Note: After calling this API, call the bottom line setting API if necessary, or the bottom line color will be overridden by the default color #e1e1e1.
*
* @param color The color to be displayed.
* @param translucent Whether to be transparent.
*
*/
- (void)setNavigationBarStyleWithColor:(UIColor *)color translucent:(BOOL)translucent;
/**
* There may be a separation line under the navigation bar, and the UI may fail to meet some UI requirements. Call this method to set the color of the separation line to prevent it from being recognized.
* Note: If you have defined the background of navigation bar, for example, by calling setNavigationBarStyleWithColor or rewriting opaqueNavigationBarColor, call this API after changing the background color.
* Otherwise, the bottom line color will be overridden by the default color #e1e1e1.
*/
- (void)setNavigationBarBottomLineColor:(UIColor*)color;
/**
* Before calling the system methods setBarTintColor, setBackGroundImage, and setBackgroundColor to set the color of navigation bar, call this method to eliminate the default effect.
* Otherwise, the color will be overlaid with the default color and cause a color deviation.
*/
- (void)resetNavigationBarColor;
/**
*
* To prevent the issue that the navigation bar flashes when a user swipes right to go back or cancel an operation, do not call this method.
*/
- (void)setNavigationBarMaskLayerWithtColor:(UIColor *)color;
/**
* Return the current background color of the navigation bar.
*
* @return Return the current background color of the navigation bar.
*/
- (UIColor*)getNavigationBarCurrentColor;
@end
Sample code
// Initialize UINavigationController.
UINavigationController *navBar = [[UINavigationController alloc] initWithNavigationBarClass:NSClassFromString(@"AUNavigationBar") toolbarClass:nil];
// Configure the navigation bar in VC.
AUBarButtonItem *cancelItem = [AUBarButtonItem backBarButtonItemWithTitle:@"Back" target:self action:@selector(cancel)];
cancelItem.backButtonTitle = @"Cancel";
self.navigationItem.leftBarButtonItem = cancelItem;
UIImage *image1 = [AUIconView iconWithName:kICONFONT_MAP width:22 color:AU_COLOR_LINK];
UIImage *image2 = [AUIconView iconWithName:kICONFONT_HELP width:22 color:AU_COLOR_LINK];
AUBarButtonItem *rightItem1 = [[AUBarButtonItem alloc] initWithImage:image1 style:UIBarButtonItemStylePlain target:self action:@selector(rightBarItemPressed)];
AUBarButtonItem *rightItem2 = [[AUBarButtonItem alloc] initWithImage:image2 style:UIBarButtonItemStylePlain target:self action:@selector(rightBarItemPressed)];
self.navigationItem.rightBarButtonItems = @[rightItem1, rightItem2];