In this article, we will show you how to build a basic Spring Boot based project on Alibaba Cloud using ArangoDB as the database engine.
ArangoDB is a multi-model NoSQL database management system allowing to build high performance applications using a convenient SQL-like query language or JavaScript extensions.
Previously, we've seen how to install ArangoDB (server and client) into an Alibaba Cloud Elastic Compute Service (ECS) Ubuntu instance and experimented with the command-line and graphical tools to perform some operations on databases, collections and users. Let's consider we've that base to better follow the current write-up which will show a basic API building using ArangoDB as database engine into a Spring-Boot based project.
We'll be building a simple API using Java/Spring resources. Here are some important dependencies
Maven will be used as build automation to configure our project and setup the dependencies.
Let's visit https://start.spring.io to generate the structure of a Spring Boot project with the Web dependency as shown below:
In this tutorial, we will cover the initial setup of OrientDB while building a Spring-Boot API with it on an Alibaba Cloud ECS instance.
OrientDB is an open source Java Multi-Model NoSQL database management technology that supports Graph, Document, Key-Value, GeoSpatial and Reactive models while managing queries with the well-known SQL syntax.
In this article, we'll cover some initial setup of OrientDB while building a Spring-Boot API with it on and Alibaba Cloud Elastic Compute Service (ECS) instance.
First, we need to create an ECS. For the sake of the demo, I will be using an Ubuntu one with 1 Core and 0.5 GB of memory. Log in via ssh/console as described into this guide:
Next, we need to install the available binary package, let's download the latest stable release of OrientDB (3.0.8 at the time of writing this article) corresponding to our operating system.
The command to use will be similar to this:
curl https://s3.us-east-2.amazonaws.com/orientdb3/releases/3.0.8/orientdb-3.0.8.tar.gz --output orientdb-3.0.8.tar.gz
Once downloaded, the zipped file called orientdb-3.0.8.tar.gz will be in the directory where you typed the curl command.
Now, we need to unzip that file and move its content to an opportune directory under the environment variable ORIENTDB_HOME. Here are the corresponding commands according to the current version: `
tar -xvzf orientdb-3.0.8.tar.gz to unzip the folder,
cp -r orientdb-3.0.8 /opt
##[Spring Boot with Alibaba Cloud Object Storage Service](https://www.alibabacloud.com/blog/spring-boot-with-alibaba-cloud-object-storage-service_594905)
In this article, we'll perform some basic OSS operations into a spring boot application using Alibaba Cloud's SDK interface wrapped on top of a spring dependency.
Alibaba Cloud [Object Storage Service](https://www.alibabacloud.com/product/oss) (OSS) is an encrypted storage service that allows you to safely save, back up, and archive any volume of data in the cloud. OSS is cost-effective, highly secure while being a very reliable solution for any use. It's possible to interact with it using a restful API.
In this article, we'll show how to do some basic OSS operations into a spring-boot application using an [SDK interface](https://mvnrepository.com/artifact/com.aliyun.oss/aliyun-sdk-oss) provided by Alibaba Cloud wrapped on top of a spring dependency.
###Connect to OSS
To perform any operation on our OSS we first need to connect ourselves giving us the required permissions to perform authenticated actions from our application.
It's important to create an Alibaba Cloud account to proceed with the other steps. If you don't have one already, go ahead and create your account through this link.
Let's assume that we have a fully working account. Now let's head on to the most important step, which is getting our unique credentials.
On the top bar of our dashboard, there is our profile pic on the very right side just hover it we have listed more menu items where we'll be selecting AccessKey as illustrated in the following image:
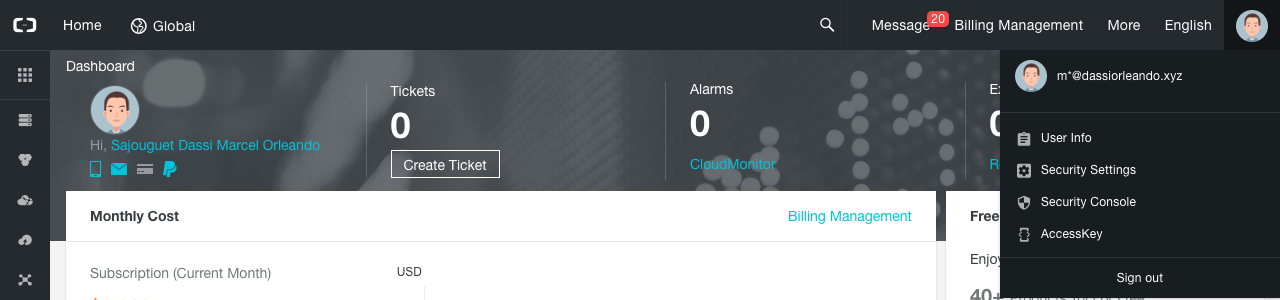
##[Deploy Spring Boot or Spring Cloud Applications to Alibaba Cloud in Eclipse](https://www.alibabacloud.com/blog/deploy-spring-boot-or-spring-cloud-applications-to-alibaba-cloud-in-eclipse_595015)
This article will show you how to deploy a Spring Boot or Spring Cloud application to Alibaba Cloud in Eclipse.
Spring Cloud and Spring Boot are currently the most popular microservice development frameworks. This article will show you how to deploy a Spring Boot or Spring Cloud application to Alibaba Cloud in Eclipse.
###Develop an Application Locally
The coding method is similar no matter whether you compile Spring Boot applications that run on the cloud or locally. Therefore, this article takes the Spring Boot application for printing "Hello World" on a Web page as an example to explain the deployment method. In this example, the built-in Tomcat container is started to process the HTTP request and print the string "Hello World" on a Web page.
The built-in Tomcat listens to requests from the root directory
###Install a Plug-in
Alibaba Cloud provides an Eclipse-based plug-in to help developers efficiently deploy applications written in the local IDE to ECS instances.
URL of the plug-in: https://www.aliyun.com/product/cloudtoolkit_en
The installation process of this Eclipse-based plug-in is similar to that of a common plug-in, and therefore will not be detailed here.
###Configure the Plug-in Preferences
After installing the plug-in, configure the preferences by choosing:
Top menu > Window > Preferences > Alibaba Cloud Toolkit > Accounts.
When the following page is displayed, configure the AK and SK of your Alibaba Cloud account to complete the configuration of preferences.( If you are using a RAM user account, enter the AK and SK of the RAM user.)
##Related Products
###[Elastic Compute Service](https://www.alibabacloud.com/product/ecs)
Elastic Compute Service is an Advanced Computing Performance to power your cloud applications and achieve faster results with low latency.
###[Simple Application Server](https://www.alibabacloud.com/product/swas)
Simple Application Server is a single server-based service for application deployment, security management, O&M monitoring, and more.
##Related Documentation
###[Use Spring Boot to develop Dubbo microservice-oriented applications](https://www.alibabacloud.com/help/doc-detail/99301.html)
Spring Boot simplifies the configuration and deployment of microservice-oriented applications. Nacos provides the service registration and discovery as well as configuration management features. Using Spring Boot and Nacos together can help you improve development efficiency. This topic describes how to use Spring Boot annotations to develop a sample Dubbo microservice-oriented application based on Nacos.
###[Spring Boot Integration](https://www.alibabacloud.com/help/doc-detail/60387.html)
Spring Cloud ACM incorporates the Health Check of Spring Boot. Access the health endpoint to see if the Spring Boot application is properly connected to the ACM server:
j{
"status": "UP",
"acm": {
"status": "UP",
"dataIds": [
"com.alibaba.cloud.acm:sample-app.properties"
]
},
"diskSpace": {
"status": "UP",
"total": 1000240963584,
"free": 858827423744,
"threshold": 10485760
},
"refreshScope": {
"status": "UP"
}
}`
Understand the advantage of a cloud server. Learn how to purchase ECS on Alibaba Cloud, manage the server on our console, backup critical data and ensure your system can adjust according to business needs.
For the security measures of the host on the cloud, we need to consider more factors, such as the configuration of the firewall inside the host, as well as various settings related to operating system user management and privilege management. Only by understanding and correctly configuring the security settings inside these hosts you can better cooperate with various cloud security products. This course is designed to help you better understand these security setup and learn how to properly configure them to maximize the security hardening of hosts on the cloud.
Infrastructure Transition to DevOps with Alibaba Cloud - Part 1: Container Services
2,600 posts | 754 followers
FollowAlibaba Clouder - June 5, 2019
Alibaba Clouder - November 26, 2018
Alibaba Developer - August 18, 2020
Alibaba Cloud Community - May 6, 2024
Alibaba Clouder - December 15, 2020
Alibaba Developer - July 8, 2021
2,600 posts | 754 followers
FollowRespond to sudden traffic spikes and minimize response time with Server Load Balancer
Learn MoreA scalable and high-performance content delivery service for accelerated distribution of content to users across the globe
Learn MoreMore Posts by Alibaba Clouder