This topic describes how to configure lifecycle hooks for function instances in a Node.js runtime environment.
Background information
After you configure a lifecycle hook for a function instance, Function Compute invokes the hook when a related lifecycle event for the instance occurs. The following lifecycle hooks can be configured for a Node.js runtime environment: Initializer, PreFreeze, and PreStop. For more information, see Function instance lifecycle.
The billing rules for the lifecycle hooks of function instances are the same as the billing rules for common invocation requests. However, the execution logs can be queried only in Function Logs, Instance Logs, and Advanced Logs. The logs for lifecycle hooks are not displayed in Call Request List. For more information, see View the logs of instance lifecycle hooks..
Initializer hooks
An Initializer hook is executed after a function instance is started and before a handler runs. Function Compute ensures that an Initializer hook is successfully executed at most once in the lifecycle of a function instance. For example, if your Initializer hook fails to be executed, the system retries the Initializer hook until the Initializer hook is successfully executed, and then runs your handler.
An Initializer hook consists of the context input parameter and can be used in the same manner as a handler.
The following sample code provides an example on a simple Initializer hook:
exports.initialize = function(context, callback) {
console.log('initializer');
callback(null, "");
};
initialize
is the method name of an Initializer hook. The name must be the same as the value
of the Initializer Hook parameter that you configured in the Function Compute console. For example, if the value of the Initializer Hook parameter for the function is index.initialize
, Function Compute loads the initialize
method that is defined in index.js
after the Initializer hook is configured.
Method signature
- The input parameter
context
specifies the runtime context provided when the FC function is invoked. - No value is returned.
PreFreeze hooks
A PreFreeze hook is executed before a function instance is frozen. The method signature of a PreFreeze hook is the same as the method signature of an Initializer hook.
The following sample code provides an example on a simple PreFreeze hook:
module.exports.preFreeze = function(context, callback) {
console.log('preFreeze');
callback(null, "");
};
PreStop hooks
A PreStop hook is executed before a function instance is destroyed. The method signature of a PreStop hook is the same as the method signature of an Initializer hook.
The following sample code provides an example on a simple PreStop hook:
module.exports.preStop = function(context, callback){
console.log('preStop');
callback(null, "");
}
Configure lifecycle hooks
Use the Function Compute console
[File name.Method name]
.
- If you set Initializer Hook to
index.initialize
, theinitialize
method in theindex.js
file is used. - If you set PreFreeze Hook to
index.preFreeze
, thepreFreeze
method in theindex.js
file is used. - If you set PreStop Hook to
index.preStop
, thepreStop
method in theindex.js
file is used.
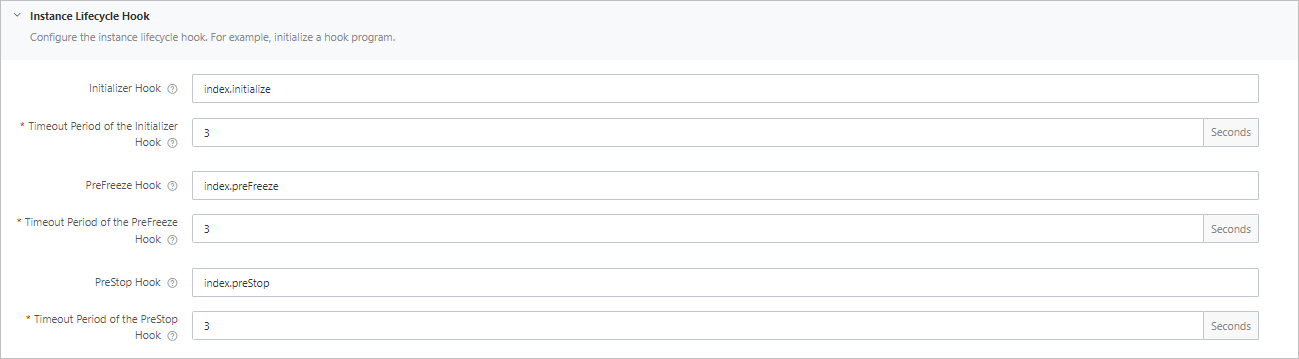
Use Serverless Devs
s.yaml
file.
- Configure the Initializer hook
Add the initializer and initializationTimeout fields to the function parameter.
- Configure the PreFreeze hook
Add the instanceLifecycleConfig.preFreeze field, including handler and timeout, to the function parameter.
- Configure the PreStop hook
Add the instanceLifecycleConfig.preStop field, including handler and timeout to the function parameter.
Sample code:
edition: 1.0.0 # The version of the YAML syntax. The version complies with the semantic versioning specifications.
name: hello-world # The name of the project.
access: default # The alias of the key.
services:
fc-deploy-test: # The name of the module.
component: devsapp/fc # The name of the component.
props: # The property value of the component.
region: cn-hangzhou # The ID of the region.
service: # The configurations of the service.
name: fc-deploy-service # The name of the service.
description: dem component # A brief description of the service.
function: # The configurations of the function.
name: fc-base-service # The name of the function.
description: 'this is test' # A brief description of the function.
codeUri: './code' # The directory where the code is located.
handler: 'index.handler' # The handler of the function. The format varies based on the programming language.
memorySize: 128 # The memory size of the function.
runtime: nodejs14 # The runtime environment.
timeout: 60 # The timeout period for the execution of the function.
initializationTimeout: 20 # The timeout period for the execution of the Initializer method.
initializer: index.initialize # The Initializer method.
instanceLifecycleConfig: # The extension function.
preFreeze: # The PreFreeze function.
handler: index.preFreeze # The handler.
timeout: 60 # The timeout period.
preStop: # The PreStop function.
handler: index.preStop # The handler.
timeout: 60 # The timeout period.
For more information about the YAML syntax of Serverless Devs, see Serverless Devs commands.
View the logs of instance lifecycle hooks.
You can view the logs for lifecycle hook in Function Logs.
Sample programs
Function Compute provides a sample program on the MySQL database that uses an Initializer hook and a PreStop hook. In this example, the Initializer hook is used to obtain the MySQL database configurations from environment variables, create the MySQL database connections, and test the connectivity. The PreStop hook is used to close the MySQL connection.
For more information, see nodejs14-mysql.